Unlike ArrayList, where the elements are arranged in an array, side by side, in LinkedList, this is not the case. In LinkedList, each element contains a pointer to the next element. Elements are linked.
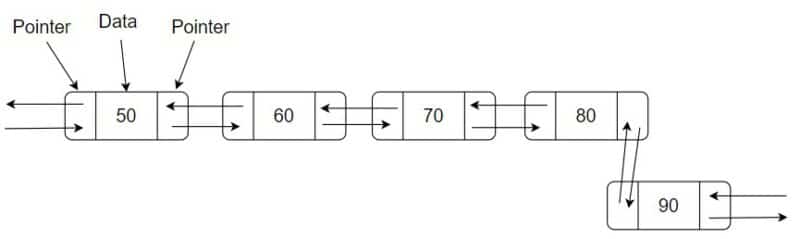
Difference between ArrayList and LinkedList
- ArrayList uses a dynamic array to store the elements internally, while LinkedList uses a doubly-linked list,
- ArrayList is better for storing and accessing data, while LinkedList is better for manipulating data,
- The ArrayList class implements the List interface only, while LinkedList implements List and Deque interfaces,
- Manipulation with ArrayList is slower because it internally uses an array.
How to create a LinkedList in Java?
We create a new LinkedList object by instantiating it, just like any other class in Java.
LinkedList<String> list = new LinkedList<>();
Between the <> brackets, we specify which type of elements the list will contain.
Or using polymorphism. The variable that holds the address of the new object will be of type List. This is possible because LinkedList implements the List interface.
List<Integer> list = new LinkedList<>();
LinkedList inherits the same methods as ArrayList, so we use exactly the same methods to manipulate elements.
How to add elements to LinkedList in Java?
We add elements using the add() method that the LinkedList class inherits from the Collection interface.
Example:
class Test { public static void main(String[] args) { List<String> list = new LinkedList<>(); list.add("Steve"); list.add("Megan"); list.add("Melissa"); list.add("Ryan"); System.out.println(list); } }
Using the addAll() method, we can add all the elements of an existing list to the newly created list.
Example:
class Test { public static void main(String[] args) { List<String> list = new LinkedList<>(); list.add("Steve"); list.add("Megan"); list.add("Melissa"); list.add("Ryan"); List<String> list2 = new LinkedList<>(); list2.addAll(list); System.out.println(list2); } }
How to remove elements from LinkedList?
We can remove list elements in three ways:
By element
We can use the remove(Object o) method and specify the exact element we want to remove from the list.
Example:
class Test { public static void main(String[] args) { List<String> list = new LinkedList<>(); list.add("Steve"); list.add("Megan"); list.add("Melissa"); list.add("Ryan"); list.remove("Megan"); System.out.println(list); } }
By index
We can use the remove(int index) method and specify the index of an element we want to remove from the list.
Example:
class Test { public static void main(String[] args) { List<String> list = new LinkedList<>(); list.add("Steve"); list.add("Megan"); list.add("Melissa"); list.add("Ryan"); list.remove(2); System.out.println(list); } }
Removing all elements that are present in the specified collection
We can use removeAll(Collection<?> c) to remove all elements present in the collection we passed in as a parameter.
class Test { public static void main(String[] args) { List<String> list = new LinkedList<>(); list.add("Steve"); list.add("Megan"); List<String> list2 = new LinkedList<>(); list2.add("Steve"); list2.add("Megan"); list2.add("Melissa"); list2.add("Ryan"); list2.removeAll(list); System.out.println(list2); } }
Clear the list / remove all elements from the list
We can use the clear() method to remove all elements from the list.
Example:
class Test { public static void main(String[] args) { List<String> list = new LinkedList<>(); list.add("Steve"); list.add("Megan"); list.add("Melissa"); list.add("Ryan"); list.clear(); System.out.println(list); } }
How to change an element in LinkedList?
We can replace the element using the set(int index, String element) method. As parameters, we pass the index of the element and the element with which we want to replace it.
Example:
class Test { public static void main(String[] args) { List<String> list = new LinkedList<>(); list.add("Steve"); list.add("Megan"); list.add("Melissa"); list.add("Ryan"); list.set(0, "Elon"); System.out.println(list); } }
How to access LinkedList elements?
To access an element of the list, we use the get(int index) method and pass the index of the element we want to fetch.
Example:
class Test { public static void main(String[] args) { List<String> list = new LinkedList<>(); list.add("Steve"); list.add("Megan"); list.add("Melissa"); list.add("Ryan"); System.out.println(list.get(3)); } }
How to get the size of the LinkedList in Java?
To find out the size of the LinkedList in Java, we use the size() method that the LinkedList class inherits from the Collection interface.
Example:
class Test { public static void main(String[] args) { List<String> list = new LinkedList<>(); list.add("Steve"); list.add("Megan"); list.add("Melissa"); list.add("Ryan"); System.out.println("Size of the list: " + list.size()); } }