Java Type Casting is the process of changing one data type into another.
This can be done in 2 ways:
- If the types are compatible, Java will perform the conversion implicitly (automatically). This is called Widening.
- If they are incompatible, then the programmer must perform explicit casting or Narrowing.
There are many Type Conversion code examples on this site if you want to learn more.
Widening
Widening is a process in which one type is automatically converted to another.
There are two cases of widening:
- When both types are compatible with each other,
- When we assign a value of a smaller data type to a bigger data type.
Example:
class Test { public static void main(String[] args) { short a = 10; int b = a; // automatically converts the short type into int type int c = 10; long d = c; // automatically converts the integer type into long type float f = d; // automatically converts the long type into float type System.out.println(a); System.out.println(b); System.out.println(c); System.out.println(d); System.out.println(f); } }
Output: 10 10 10 10 10.0
In the above example, we assigned a smaller type to a larger one, and the conversation was made automatically.
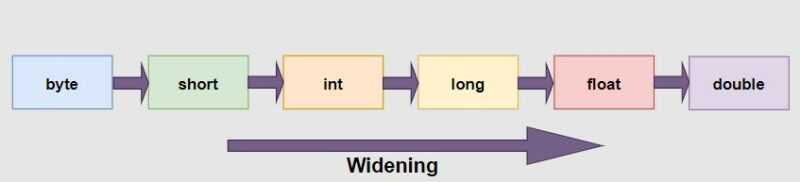
Narrowing
Converting a higher data type into a lower one is called narrowing type casting. It must be done explicitly by the programmer. Otherwise, a compiler error will occur.
Example:
class Test { public static void main(String[] args) { double d = 10.5; long b = (long) d; // converting double data type into long data type long l = 10; int a = (int) l; // converting long data type into integer data type System.out.println(d); System.out.println(b); System.out.println(l); System.out.println(a); } }
Output: 10.5 10 10 10
In the above example, we assigned a higher data type to a lower one. We did this explicitly by specifying which type we wanted to convert the value to in parentheses.
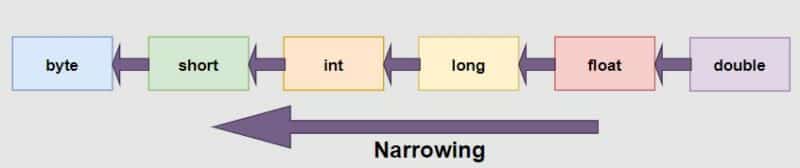
In this lesson, you learned what type casting is in Java. For more examples, check out other Conversion examples in Java.
In the next tutorial, you will learn about Upcasting vs Downcasting.
Happy learning!