In this Reactive Programming in Java tutorial, you will understand the need for Reactive programming in modern software development.
You will learn what is Reactive programming, how Reactive Streams work, what types of Publishers we have in Project Reactor, and many more.
Prerequisites
- Java 11 or higher
- Prior Java Experience – Java Tutorial for Beginners
- Experience with Functional features sush as Lambdas, Streams and Method References
- IntelliJ or any other IDE
Why Reactive Programming?
Let’s see one example of a traditional application that interacts with multiple data sources:
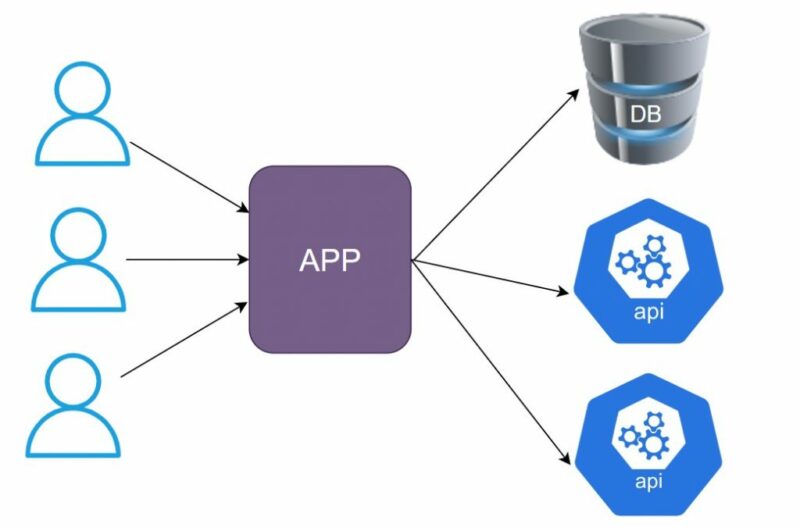
In the example above, we have an application that receives the request from the client (user). Let’s say the request is for login.
The user enters credentials, and the app first needs to call the database to collect the valid credentials for that user and to perform some validation by calling two external API services. Finally, when it receives the responses from both APIs, the response will be sent back to the user (either a successful or failed login message).
Can you see the issue here? Since the app is written in a synchronous fashion, we have three blocking points here. The blocking points are interactions with DB and two external API services.
The latency of the request from the client would be the summation of (DB + API + API) response times. This means that the thread will be blocked waiting for the action to complete (retrieving the data from DB) to continue with the next task (executing the API call).
It can become a big issue when the application has multiple users simultaneously. Not to mention modern apps that can have more than 10000 concurrent users.
Most of today’s applications have thread pools to deal with such a problem. Still, threads are expensive resources, and due to various limitations, it is impossible to have the number of threads that matches the number of users supported through the application.
We do have the Asynchronous (Parallel) APIs in Java that we can use, and those are Callbacks and Completable Futures, but both have some disadvantages.
Callbacks
Callbacks are asynchronous methods that accept a callback as a parameter and invoke it when the blocking call completes.
Writing code with Callbacks is hard and difficult to read and maintain.
Completable Futures
Completable Futures got introduced as part of Java 8 and it allows us to write the code in a functional style. Using it we can easily combine multiple asynchronous computations.
The main disadvantage of the Completable Future is that it does not handle well the async computations that return the collection of some data.
Conclusion
When it comes to huge volumes of data or serving many concurrent users, we need asynchronous processing to make our systems fast and responsive. Current Async APIs in Java have some limitations, so the Java world is moving to the new programming paradigm: Reactive Programming.
In the upcoming lessons, you will see the main advantages of writing the code in a Reactive manner.
Let’s start with the lessons.
Happy coding!
Reactive Programming Tutorials
- Introduction to Reactive Programming
- Introduction to Reactive Streams in Java
- Project Reactor in Java
- Create a Mono in Java Reactor
- Subscribe to a Mono in Java Reactor
- Extract data from Mono in Java
- Create a Flux in Java Reactor
- Subscribe to Flux in Java Reactor
- Extract data from Flux
- Convert Mono to Flux and vice versa
- How Mono and Flux work internally?
- Transform Flux and Mono using Operators
- Combine Flux and Mono Publishers
- doOn Callbacks in Project Reactor
- Handling exceptions in Project Reactor
- Retry failed operation in Project Reactor
- Hot and Cold Publishers in Project Reactor
- Reactor Execution Model – Threading and Schedulers
- subscribeOn and publishOn operators in Project Reactor
- Implementing Backpressure in Project Reactor
- Testing with StepVerifier in Project Reactor