In this tutorial, you will learn how to create and customize UITextView programmatically in Swift.
Creating and customizing a UITextView
programmatically in Swift involves several steps. Here’s how you can do it:
Step 1: Create UITextView Programmatically
To create UITextView programmatically, you will need to first create an instance of UITextView
. This can be done by instantiating the UITextView
object. Declare your UITextView
at the class level of the view controller it’s used in.
let textView = UITextView()
This line of code creates an instance of UITextView
named textView
. You can replace textView
with any name you prefer.
Step 2: Set UITextView Frame
Next, you need to set the frame of the UITextView
. A frame is a rectangle that defines the size and position of a view.
textView.frame = CGRect(x: 20.0, y: 90.0, width: 250.0, height: 250.0)
This code sets the UITextView
‘s position (x: 20.0, y: 90.0) and size (width: 250.0, height: 250.0). You can adjust these values according to your needs.
Step 3: Center UITextView
To center the UITextView
within the view, you can set the center of the UITextView
to be the center of the view.
textView.center = self.view.center
This line of code centers the UITextView
horizontally and vertically within the view.
Step 4: Change UITextView Text Color
To change the text color of the UITextView
, use the textColor
property.
textView.textColor = UIColor.blue
This code sets the text color of the UITextView
to blue. You can replace UIColor.blue
with any color you prefer.
Step 5: Change UITextView Background Color
To change the background color of the UITextView
, use the backgroundColor
property.
textView.backgroundColor = UIColor.lightGray
This code sets the background color of the UITextView
to light gray. You can replace UIColor.lightGray
with any color you prefer.
Step 6: Set UITextView Font Size and Font Color
To update UITextView
font size and color, use the font
property.
textView.font = UIFont.systemFont(ofSize: 20) textView.textColor = UIColor.white
This code sets the font size of the UITextView
to 20 and the text color to white.
Step 7: Set UITextView Font Style
To set the font style, use the font
property again.
textView.font = UIFont.boldSystemFont(ofSize: 20) textView.font = UIFont(name: "Verdana", size: 17)
This code first sets the font to bold system font of size 20, and then changes the font to Verdana of size 17.
Step 8: Enable and Disable UITextView Editing
To enable or disable editing, use the isEditable
property.
// Make UITextView Editable textView.isEditable = true
This code makes the UITextView
editable. If you want to disable editing, set isEditable
to false
.
Step 9: Capitalize UITextView Text
To capitalize all character’s user types, use the autocapitalizationType
property.
// Capitalize all characters user types textView.autocapitalizationType = UITextAutocapitalizationType.allCharacters
This code capitalizes all characters that the user types.
Step 10: Make UITextView Web Links Clickable
To make web links clickable, set the isSelectable
property to true
and the dataDetectorTypes
property to .link
.
// Make UITextView web links clickable textView.isSelectable = true textView.isEditable = false textView.dataDetectorTypes = UIDataDetectorTypes.link
This code makes the web links in the UITextView
clickable.
Step 11: Round UITextView Corners
To round the corners of the UITextView
, use the layer.cornerRadius
property.
// Make UITextView corners rounded textView.layer.cornerRadius = 10
This code rounds the corners of the UITextView
with a radius of 10.
Step 12: Enable Auto-Correction and Spellcheck
To enable auto-correction and spellcheck, use the autocorrectionType
and spellCheckingType
properties.
// Enable auto-correction and Spellcheck textView.autocorrectionType = UITextAutocorrectionType.yes textView.spellCheckingType = UITextSpellCheckingType.yes
This code enables auto-correction and spellcheck for the UITextView
.
Step 13: Add UITextView to the View
Finally, after creating and customizing the UITextView
, you need to add it to the view hierarchy so it appears on the screen. Use the addSubview(_:)
method to do this.
view.addSubview(textView)
This line of code adds the textView
instance to the current view. This makes the textView
visible on the screen because it’s now part of the view hierarchy.
Complete code example:
Here’s the complete code for creating and customizing a UITextView
using the above code snippets together:
import UIKit class ViewController: UIViewController { override func viewDidLoad() { super.viewDidLoad() let textView = UITextView(frame: CGRect(x: 20.0, y: 90.0, width: 250.0, height: 250.0)) textView.contentInsetAdjustmentBehavior = .automatic textView.center = self.view.center textView.textAlignment = NSTextAlignment.justified textView.textColor = UIColor.blue textView.backgroundColor = UIColor.lightGray textView.font = UIFont.systemFont(ofSize: 20) textView.textColor = UIColor.white textView.font = UIFont.boldSystemFont(ofSize: 20) textView.font = UIFont(name: "Verdana", size: 17) textView.isEditable = true textView.autocapitalizationType = UITextAutocapitalizationType.allCharacters textView.isSelectable = true textView.isEditable = true textView.dataDetectorTypes = UIDataDetectorTypes.link textView.layer.cornerRadius = 10 textView.autocorrectionType = UITextAutocorrectionType.yes textView.spellCheckingType = UITextSpellCheckingType.yes view.addSubview(textView) } }
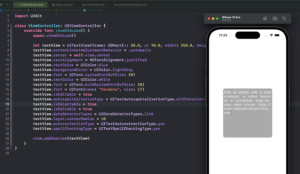
Conclusion
If you are interested in video lessons on how to write Unit tests and UI tests to test your Swift mobile app, check out this page: Unit Testing Swift Mobile App
I hope this tutorial was helpful to you. You now know how to create and customize UITextView programmatically.
To learn more about Swift and to find other code examples, check the following page: Swift Code Examples.