The Java compiler executes commands from top to bottom. However, we can control the code execution flow using Java Control Flow Statements.
There are three types of Control Flow Statements in Java:
- Decision-Making statements
- Loop statements
- Jump statements
In this tutorial, we will discuss Decision-Making statements.
Decision-Making statements
We have two types of Decision-Making statements:
- if-else Statement
- switch Statement
If-else Statement in Java
If-else controls the flow of code execution by evaluating some boolean expressions.
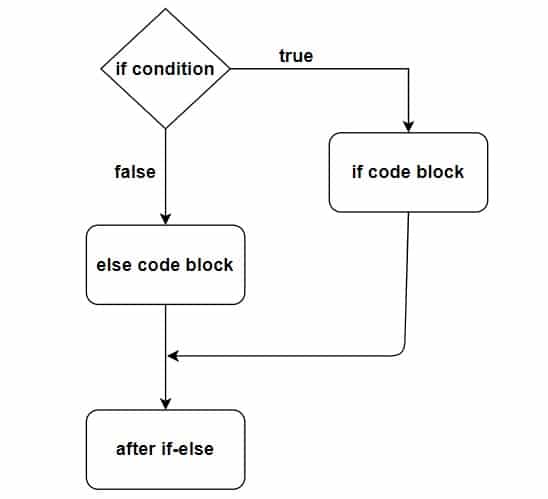
We have 4 types of if-else statements:
- if statement
- if-else statement
- else if statement
- Nested if/if-else statement
1. If Statement
The if statement forwards code execution into its block based on some boolean expression.
Example:
class Test { public static void main(String[] args) { int a = 10; int b = 5; if (a > b) { // if true, enter the if code block System.out.println("a is greater than b"); } System.out.println("Rest of the code ..."); } }
Otherwise, the if code block will be skipped, like in this example:
class Test { public static void main(String[] args) { int a = 5; int b = 10; if (a > b) { // if true, enter the if code block System.out.println("A is greater then B"); } System.out.println("Rest of the code ..."); } }
2. If-else Statement
By using the if-else statement, we will ensure that one of the two code blocks will be executed based on some boolean expression.
Example:
class Test { public static void main(String[] args) { int a = 10; int b = 5; if (a > b) { // if true, enter the "if" code block, otherwise enter the "else" code block System.out.println("A is greater than B"); } else { System.out.println("A is not greater thn B"); } System.out.println("Rest of the code ..."); } }
If a is not greater than b, then only the else block would execute, and the if block would be skipped:
class Test { public static void main(String[] args) { int a = 5; int b = 10; if (a > b) { // if true, enter the "if" code block, otherwise enter the "else" code block System.out.println("A is greater then B"); } else { System.out.println("A is not greater than B"); } System.out.println("Rest of the code ..."); } }
class Test { public static void main(String[] args) { String str = "alegrutechblog"; if (str.length() < 20) { System.out.println("The string is valid!"); } else { System.out.println("The string is valid!"); } } }
3. Else if Statement
Using else if statements, we create a chain of if-else, where the one which boolean expression evaluates to true will be executed.
Example:
class Test { public static void main(String[] args) { int number = 37; if (number > 50) { System.out.println("The number is greater than 50"); } else if (number > 40) { System.out.println("The number is greater than 40"); } else if (number > 30) { System.out.println("The number is greater than 30"); } } }
See an example when we have two else if statements that evaluate to true:
class Test { public static void main(String[] args) { int number = 37; if (number > 50) { // false System.out.println("The number is greater than 50"); } else if (number > 40) { // false System.out.println("The number is greater than 40"); } else if (number > 30) { // true System.out.println("The number is greater than 30"); } else if (number > 20) { // true System.out.println("The number is greater than 20"); } } }
We can also put one else at the end, which will be executed if none of the above statements are true.
class Test { public static void main(String[] args) { int number = 28; if (number > 50) { System.out.println("The number is greater than 50"); } else if (number > 40) { System.out.println("The number is greater than 40"); } else if (number > 30) { System.out.println("The number is greater than 30"); } else { System.out.println("The number is smaller than 30"); } } }
4. Nested if/if-else Statement
We can have nested if-else statements also.
Nested if statement:
class Test { public static void main(String[] args) { String str = "learnjava"; if (str.length() < 20) { if (str.length() == 9) { System.out.println("The length is 9"); } } else { System.out.println("Else block ..."); } } }
class Test { public static void main(String[] args) { String str = "learnjavawithalegru"; if (str.length() < 20) { if (str.length() == 9) { System.out.println("The length is 9"); } else { System.out.println("The length is smaller than 20, but not equal to 9!"); } } else { System.out.println("Else block ..."); } } }
class Test { public static void main(String[] args) { String str = "alegrutechblog"; if (str.equals("alegrutechblog")) { if (str.endsWith("tech")) { System.out.println("It ends with 'tech'. "); } else if (str.endsWith("blog")) { System.out.println("It ends with 'blog'. "); } else { System.out.println("Nested else block ..."); } } else { System.out.println("else block ..."); } } }