To schedule a task in Spring Boot we use the @Scheduled annotation. We place the @Scheduled annotation above the declaration of the method that should not expect any parameters, and the return type should be void.
Spring Boot internally uses the TaskScheduler interface to schedule the annotated execution methods.
Schedule a task in Spring Boot – steps
1. Create a simple app
Create one simple application in Spring Initializer .
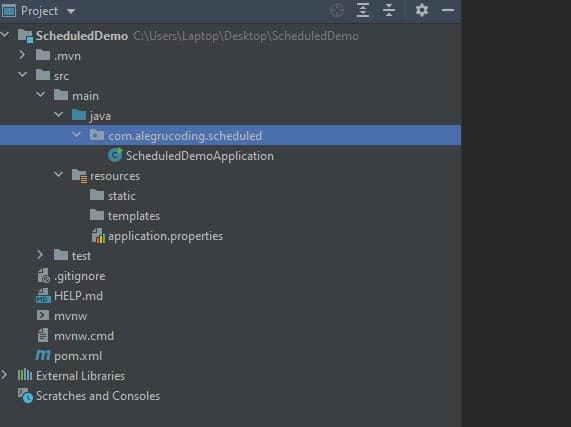
2. Enable scheduling
Enable scheduling by adding the @EnableScheduling annotation in your app’s main class.
import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; import org.springframework.scheduling.annotation.EnableScheduling; @EnableScheduling @SpringBootApplication public class ScheduledDemoApplication { public static void main(String[] args) { SpringApplication.run(ScheduledDemoApplication.class, args); } }
3. Add @Scheduled annotation
Create a service class and inside create a method that will be decorated with a @Scheduled annotation
import org.springframework.scheduling.annotation.Scheduled; import org.springframework.stereotype.Component; @Component public class ScheduledTaskService { @Scheduled(fixedRate = 5000) public void execute() { // some logic that will be executed on a schedule System.out.println("Code is being executed..."); } }
Now, let’s run the app:
Output: 2021-11-15 12:35:00.828 INFO 12408 --- [main] c.a.scheduled.ScheduledDemoApplication : Started ScheduledDemoApplication in 5.725 seconds (JVM running for 6.908) Code is being executed... Time: 15/11/2021 12:35:05 Code is being executed... Time: 15/11/2021 12:35:10 Code is being executed... Time: 15/11/2021 12:35:15 Code is being executed... Time: 15/11/2021 12:35:20
Here, we set the fixedRate = 5000 (5s), which means this method will be executed every 5 seconds.
Other types of scheduling
Schedule a Task with Fixed Delay
In the above example, we scheduled a task with a fixed rate. However, it can also be scheduled with a fixed delay (fixedDelay), which counts the delay after the completion of the last invocation.
import org.springframework.scheduling.annotation.Scheduled; import org.springframework.stereotype.Component; import java.time.LocalDateTime; import java.time.format.DateTimeFormatter; @Component public class ScheduledTaskService { private final DateTimeFormatter formatter = DateTimeFormatter.ofPattern("dd/MM/yyyy hh:mm:ss"); @Scheduled(fixedDelay = 5000) public void execute() throws InterruptedException { // some logic that will be executed on a schedule Thread.sleep(3000); System.out.println("Code is being executed... Time: " + formatter.format(LocalDateTime.now())); } }
Schedule a task with Initial Delay
The task can be scheduled with some initial delay (initialDelay), which delays the first execution of the task.
@Component public class ScheduledTaskService { private final DateTimeFormatter formatter = DateTimeFormatter.ofPattern("dd/MM/yyyy hh:mm:ss"); @Scheduled(fixedRate = 5000, initialDelay = 4000) public void execute() throws InterruptedException { // some logic that will be executed on a schedule System.out.println("Code is being executed... Time: " + formatter.format(LocalDateTime.now())); } }
Schedule a task Using the cron expression
If you need more control over scheduling then use the cron expressions.
@Component public class ScheduledTaskService { private final DateTimeFormatter formatter = DateTimeFormatter.ofPattern("dd/MM/yyyy hh:mm:ss"); @Scheduled(cron = "0 0 10 * * *") public void execute() throws InterruptedException { // some logic that will be executed on a schedule System.out.println("Code is being executed... Time: " + formatter.format(LocalDateTime.now())); } }
The above task will be executed every day at 10am.
Here are some examples of the cron expressions:
Cron Expression | Meaning |
---|---|
0 0 * * * * |
Top of every hour of every day |
*/10 * * * * * |
Every ten seconds |
0 0 8-10 * * * |
8, 9 and 10 o’clock of every day |
0 0 6,19 * * * |
6:00 AM and 7:00 PM every day |
0 0/30 8-10 * * * |
8:00, 8:30, 9:00, 9:30, 10:00 and 10:30 every day |
0 0 9-17 * * MON-FRI |
On the hour nine-to-five weekdays |
0 0 0 25 12 ? |
Every Christmas Day at midnight |
Use value from the property file
It is always better to externalize the value of the scheduled task into a property file instead of hardcoding it.
To read the value from the property file, use the “{full_path_to_property}” pattern.
Example
application.properties
com.alegrucoding.cron=*/10 * * * * *
ScheduledTaskService.class
@Scheduled(cron = "${com.alegrucoding.cron}") public void execute() throws InterruptedException { // some logic that will be executed on a schedule System.out.println("Code is being executed... Time: " + formatter.format(LocalDateTime.now())); }