Spring Boot is a popular framework for building Java-based web applications. It provides a simple and efficient way to build, deploy and run Java applications quickly.
In this tutorial, we’ll explore the framework’s basics, including its features, advantages over the Spring Framework, and how to get started with creating your first application using it. Whether you’re new to Java or looking to expand your skills, this tutorial will help you gain a better understanding of Spring Boot and how to use it to build powerful web applications.
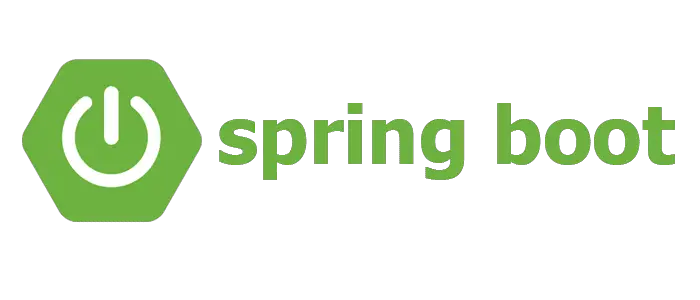
Spring Framework in Java
Spring Framework is a widely used open-source Java framework that provides a comprehensive programming and configuration model for developing enterprise-level Java applications. It is designed to create standalone, production-grade applications that run on the Java Virtual Machine (JVM).
One of the key advantages of Spring Framework is its support for creating modular applications consisting of loosely coupled components. This makes it an ideal choice for building microservices-based architectures, where different services can be developed independently and easily integrated as needed. The modular nature of Spring Framework also makes it easier to maintain and update individual components without affecting the rest of the application.
Microservices architecture is an approach in which a single application is composed of many loosely coupled and independently deployable services called microservices.
Spring Framework provides a number of features and capabilities that help developers build scalable and robust applications, including:
- Dependency Injection: Spring’s core feature is its dependency injection mechanism, which allows developers to write loosely coupled code that is easy to maintain and test.
- AOP: Spring provides support for Aspect-Oriented Programming (AOP), which allows developers to modularize cross-cutting concerns like security, logging, and caching.
- MVC: Spring provides a Model-View-Controller (MVC) framework that simplifies the development of web applications by separating the presentation layer from the business logic.
- Integration: Spring provides integration with a wide range of enterprise technologies like Hibernate, JPA, and JMS, making it easier to develop enterprise applications.
- Testing: Spring provides a number of tools and frameworks for testing applications, including the Spring Test Framework and the Spring MVC Test Framework.
In addition to these core features, Spring Framework also provides support for transaction management, caching, messaging, and more.
Overall, Spring Framework is a powerful and versatile framework that provides a wealth of features and capabilities for building Java applications. Whether you’re building a small web application or a large-scale enterprise system, Spring Framework provides the tools and support you need to be productive and successful.
What is Spring Boot?
Spring Boot is a framework for building Java web applications that is built on top of the popular Spring Framework. It makes it easier to create stand-alone, production-grade Spring-based applications that you can run with minimal setup.
With Spring Boot, you can get started quickly with a pre-configured Spring environment that includes everything you need to build a web application, including web servers, templating engines, and more. It is designed to take the complexity out of building Java web applications, allowing you to focus on writing your application’s business logic instead of configuring infrastructure.
Spring Boot Features
Spring Boot includes many features that make it a popular choice for Java web development. Here are some of its key features:
- Auto-Configuration: Spring Boot provides a large number of pre-configured components that are automatically configured based on the classpath, making it easy to get started quickly. For example, if you include the
spring-boot-starter-web
dependency in your project, a web server will be automatically configured, as well as a templating engine, and other components that you need to build a web application.<dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency>
- Embeddable Web Server: Spring Boot includes an embedded Tomcat, Jetty or Undertow web server, which makes it easy to run your web application without having to set up a separate web server.
- Actuator: Spring Boot provides an Actuator that allows you to monitor and manage your application. You can use it to view application metrics, manage caches, and more. For example, the
/health
endpoint provides information about the health of your application, and the/metrics
endpoint provides information about various metrics, such as the number of requests processed by your application. - Spring CLI: Spring Boot provides a command-line interface that allows you to quickly create, test, and run applications from the command line. For example, you can create a new project using the following command:
spring init --dependencies=web my-project
- Production-ready: Spring Boot is designed to be production-ready. It includes features like metrics, health checks, and externalized configuration that are essential for running applications in production. For example, you can use its externalized configuration feature to configure your application using properties files, YAML files, or environment variables.
server: port: 8080 spring: datasource: url: jdbc:mysql://localhost/mydb username: myuser password: mypass
Why Use Spring Boot?
There are many reasons why Java developers choose to use Spring Boot for building web applications. Here are some of the key benefits:
- Easy to get started: It is much easier to get started with building web applications. With the auto-configuration feature, you can get a working web application up and running in just a few minutes.
- Reduced boilerplate code: The amount of boilerplate code that you need to write is reduced, allowing you to focus on writing your application’s business logic.
- Faster development: Pre-configured components and out-of-the-box features make it faster to develop web applications, reducing the amount of time and effort required to build a complete application.
- Robust and scalable: Spring Boot is designed to be robust and scalable, with features like Actuator, externalized configuration, and built-in health checks that make it easy to monitor and manage your application.
- Large community: A large and active community of developers and users, which means that there are plenty of resources and support available if you need help.
Spring Boot vs. Spring Framework
When it comes to building Java applications, Spring Framework and Spring Boot are two of the most popular frameworks in use today. While they share a common core, they are designed to accomplish different things.
Spring Framework
Spring Framework is a powerful, feature-rich framework for building enterprise-grade Java applications. It provides a wide range of modules, including the core container, data access, web, and messaging, that can be used to build almost any kind of application.
One of the key features of Spring Framework is its Inversion of Control (IoC) container, which allows developers to manage dependencies and component lifecycles. Spring Framework also provides a powerful set of tools for data access, such as the Spring Data module, which can be used to interact with databases and other data stores.
Spring Boot
Spring Boot, on the other hand, is an opinionated framework designed to make it easy to create Spring-based applications quickly and with minimal configuration. It builds on top of Spring Framework and includes many of its features, such as the IoC container and Spring Data.
One of the key benefits of Spring Boot is its auto-configuration feature, which automatically configures the application based on the dependencies it finds on the classpath. For example, if the application includes Spring Data, a DataSource and EntityManagerFactory will be automatically configured.
Which one to choose?
So, which one should you choose? It depends on your needs. If you need a lot of flexibility and control over your application, Spring Framework might be the better choice. On the other hand, if you want to get up and running quickly and with minimal configuration, Spring Boot is a great option. Here are some additional factors to consider when deciding between these two frameworks:
Project Complexity
If you’re working on a large, complex project that requires a high degree of flexibility and customization, Spring Framework might be the better choice. Spring Framework’s modular architecture allows you to pick and choose the components you need and configure them to suit your specific requirements. This can be a great advantage when dealing with complex business logic, data access, and integration with third-party systems.
On the other hand, if you’re building a small to a medium-sized project or a microservice, Spring Boot can be a better choice. Its opinionated approach and auto-configuration features can help you get up and running quickly with minimal configuration. This can be particularly useful for prototyping and getting your application to market faster.
Development Speed
Another factor to consider is development speed. Spring Boot’s auto-configuration and starter dependencies can save a lot of time and effort when setting up a new project. You can get a basic project up and running in minutes, which can be a huge advantage when working on tight deadlines or in a fast-paced development environment.
On the other hand, Spring Framework provides more flexibility and control, which can be beneficial in some cases but can also slow down development time. If you need to customize your application extensively or integrate with complex systems, you may find that Spring Framework provides a better fit for your needs.
Community Support
Both Spring Boot and Spring Framework have large and active communities, which means that you can find plenty of resources and support online. However, the former has gained a lot of popularity in recent years and has a particularly strong community. This can be a significant advantage when it comes to finding answers to questions, resolving issues, and getting help with development.
Skillset and Experience
Finally, it’s worth considering your team’s skillset and experience when making the descision. If your team is already familiar with Spring Framework and has experience working with it, it may be more efficient to continue using it for new projects. Similarly, if you have a team with a lot of experience in Java development but less familiarity with Spring, Spring Framework might be a better fit.
In general, Spring Boot is a great choice for building microservices and other lightweight applications, while Spring Framework is better suited for larger, more complex applications. However, both frameworks are powerful tools that can be used to build a wide range of applications, and the choice ultimately depends on the specific needs and requirements of your project.
Spring Boot Starters
Spring Boot Starters are a collection of pre-configured dependencies that make it easier to set up a project. By including starters in your project, you can quickly get up and running with various features and frameworks, without the need to manually configure everything.
Spring Boot provides several starter modules, each designed to support a specific technology or framework. Here are some of the most common starters you might use:
spring-boot-starter-web
: includes everything needed to build a web application, including Tomcat, Spring MVC, and Jackson for JSON processing.spring-boot-starter-data-jpa
: includes everything needed for working with JPA and Hibernate, including a connection pool, transaction management, and Spring Data JPA.spring-boot-starter-security
: includes everything needed for basic authentication and authorization, including Spring Security, OAuth2, and JWT support.spring-boot-starter-test
: includes everything needed for testing your application, including JUnit, Mockito, and Spring Test.
To use a starter, simply add it to your project’s dependencies in your pom.xml
or build.gradle
file. Here’s an example using the spring-boot-starter-web
starter:
<!-- pom.xml --> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> <version>2.6.2</version> </dependency>
// build.gradle dependencies { implementation 'org.springframework.boot:spring-boot-starter-web:2.6.2' }
When you add a starter to your project, Spring Boot will automatically configure the necessary beans and properties for that module. This means that you don’t need to write any boilerplate code or configuration files to get started with that technology.
For example, if you add the spring-boot-starter-web
starter to your project, Spring Boot will automatically configure a DispatcherServlet
, a ContentNegotiatingViewResolver
, and a HandlerMapping
bean. You can then create a controller and start building your application:
@RestController public class HelloController { @GetMapping("/hello") public String hello() { return "Hello, World!"; } }
By default, port 8080 will be used for your application. You can run your application using the mvn spring-boot:run
command, or by running the main method of your @SpringBootApplication
class.
That’s it! With just a few lines of code and a single starter dependency, you’ve built a working web application with Spring Boot.
Creating Your First Spring Boot Application
This tutorial, Create a Web Service Project with Spring Boot Initializr explores the process of creating a web service project using Spring Boot Initializr. It highlights multiple ways to create a web service project and focuses on creating a RestController, building and running the web service, and sending an HTTP GET request using CURL. The tutorial also provides a video tutorial to supplement the written instructions, don’t hesitate to check it out!
Conclusion
In conclusion, Spring Boot is a robust framework for building Java-based web applications. It offers several advantages over the Spring Framework, including rapid application development, simple configuration, and easy deployment. With the help of Spring Boot Starters, developers can quickly build applications without worrying about configuring the required dependencies manually.
This tutorial covered the basics of Spring Boot, including its features and how to create your first application using it. By following the steps outlined in this tutorial, you should now have a good understanding of how to get started and build powerful web applications. Make sure to check out the RESTful Web Services with Spring Boot REST page for more related tutorials.
Frequently Asked Questions
- Can I use Spring Boot with other programming languages besides Java?
No, Spring Boot is a framework built specifically for Java. Therefore, it can only be used with the Java programming language. - Can I use Spring Boot to build microservices?
Yes, Spring Boot is well-suited for building microservices. In fact, Spring Boot provides several features and tools that make it easier to build microservices, such as Spring Cloud, which offers features like service discovery, circuit breakers, and distributed tracing. Additionally, Spring Boot’s lightweight architecture and easy deployment make it an excellent choice for building microservices that can be scaled independently. Overall, Spring Boot is a popular choice for building microservices due to its flexibility, ease of use, and rich set of features. - How do I perform database operations in a Spring Boot application?
To perform database operations in a Spring Boot application, you can use Spring Data JPA. This module provides a high-level abstraction over JDBC, allowing you to interact with the database using Java objects rather than writing SQL queries. You can define your data models using JPA entities, and Spring Data JPA will generate the appropriate SQL statements to perform CRUD (Create, Read, Update, Delete) operations on the database. - Can I use Spring Boot to build both web-based and non-web-based applications?
Yes, Spring Boot can be used to build both web-based and non-web-based applications. Although Spring Boot is commonly used to build web applications, it can also be used to develop command-line applications, batch applications, and standalone applications. In fact, Spring Boot’s auto-configuration and dependency management features make it an excellent choice for building a wide range of applications in various domains. - Can I integrate Spring Boot with other third-party libraries and frameworks?
Yes, Spring Boot allows you to easily integrate with third-party libraries and frameworks. Spring Boot’s auto-configuration feature automatically configures your application based on the classpath dependencies, including third-party libraries. This feature reduces the amount of configuration required by developers and speeds up the development process. Additionally, Spring Boot provides a comprehensive set of APIs that allow you to integrate with other frameworks, such as Hibernate, JPA, and Thymeleaf, among others. With these APIs, you can easily configure and customize your Spring Boot application to meet your specific requirements. - Can I use Spring Boot to build applications for mobile platforms?
Yes, it is possible to use Spring Boot to build applications for mobile platforms. However, Spring Boot is primarily designed for building web-based applications and RESTful services. To build mobile applications, developers usually use mobile-specific frameworks or platforms, such as Android SDK, iOS SDK, or Xamarin. However, Spring Boot can be used in conjunction with these platforms to provide server-side functionality and backend services.