In this tutorial, you will learn how to setup Eureka Cluster on your local computer. Although in this tutorial, I am going to setup Eureka Cluster on my local computer, you will still learn what you need to do to make Eureka run on remote server as well.
What is Eureka Server Cluster?
A Eureka Server Cluster, also known as a Eureka Peer Awareness system, is a group of Eureka Servers that communicate with each other. This is not your average group of servers, though. They have a knack for talking to each other, sharing information about the registered services. This means even if one of the servers goes down, the others can still provide the necessary service information, ensuring high availability and fault tolerance. This is a key feature, making Eureka Server Clusters an excellent choice for large-scale systems where downtime can be costly.
Now, you may wonder why we need such a setup. Well, think of it as a team of librarians who always keep each other updated about the books in a vast library. If one of them is absent, the others can still guide you to your book. Similarly, Eureka Server Clusters ensure your microservices can always find each other, even in the case of server failures. It’s their teamwork that makes your microservices architecture robust and resilient.
In the next sections, I will guide you through setting up your own Eureka Server Cluster. So, stay tuned and get ready to learn more!
Creating Eureka Server Project
The first thing we need to do is create a new Spring Boot project. For this, we’ll use the Spring Initializr tool. It’s a fantastic tool that can quickly bootstrap our project. When creating the project, we need to make sure to add an important dependency called “Eureka Server“. This dependency will transform our ordinary Spring Boot project into a Eureka Server. Don’t worry if you’re not sure about how to do this right now. We’ll look at the details in the next step.
Required Maven Dependency
If you have created your project using Spring Initializr tool and have added Eureka Server dependency, you should see the following dependency in the pom.xml file of your project.
<dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-netflix-eureka-server</artifactId> </dependency>
In my case a complete pom.xml file looks like this:
<?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <parent> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-parent</artifactId> <version>3.1.0</version> <relativePath /> <!-- lookup parent from repository --> </parent> <groupId>com.appsdeveloperblog.tutorials</groupId> <artifactId>EurekaServerOne</artifactId> <version>0.0.1-SNAPSHOT</version> <name>EurekaServerOne</name> <description>Demo project for Spring Boot</description> <properties> <java.version>17</java.version> <spring-cloud.version>2022.0.3</spring-cloud.version> </properties> <dependencies> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-netflix-eureka-server</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-test</artifactId> <scope>test</scope> </dependency> </dependencies> <dependencyManagement> <dependencies> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-dependencies</artifactId> <version>${spring-cloud.version}</version> <type>pom</type> <scope>import</scope> </dependency> </dependencies> </dependencyManagement> <build> <plugins> <plugin> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-maven-plugin</artifactId> </plugin> </plugins> </build> </project>
Enabling Eureka Server with @EnableEurekaServer
Next, we need to add a special touch to our main application file – the one with the public static void main(String[] args)
method. We need to annotate this class with @EnableEurekaServer
. But what does this annotation do? Well, it’s like giving our project the final set of instructions to become a Eureka Server. It activates the Netflix Eureka Server related features within our Spring Boot project, allowing other microservices to register themselves, be discovered, and communicate with each other.
Your code will look something like this:
package com.appsdeveloperblog.tutorials.EurekaServerOne; import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; import org.springframework.cloud.netflix.eureka.server.EnableEurekaServer; @SpringBootApplication @EnableEurekaServer public class EurekaServerOneApplication { public static void main(String[] args) { SpringApplication.run(EurekaServerOneApplication.class, args); } }
Configure Eureka Cluster: Peer Awareness
Now that we’ve created our Eureka Server project, our next step is to configure it to act as a Eureka Discovery server. Moreover, we’ll also prepare it to run in a cluster.
Let’s envisage a scenario where we want three Eureka Servers to operate in a cluster. To configure our Eureka Server Spring Boot project to function within this cluster of three servers, we’re going to create three separate Spring Boot profiles, one for each Eureka Server instance.
What is Peer Awareness?
“Peer Awareness” is the ability of one server to know about its siblings, its peers, in a cluster. Think of it like having a group of friends. If you’re aware of what your friends are doing, where they are, or how they are doing, we say you are “peer aware”. Similarly, in a Eureka Server cluster, if one server knows about the presence and status of other servers, it is said to have “peer awareness”.
Why is this important? Imagine you’re in a team working on a project. If one team member is absent, the others should be aware of this and pick up their responsibilities to ensure the project doesn’t suffer. In the same way, if one Eureka Server goes down, the other servers in the cluster, thanks to peer awareness, will know about this. They can then step in and ensure that the service discovery and registration functionalities continue uninterrupted. This makes your system highly resilient and reliable.
Define each Peer in a hosts file
If you’re planning to run your Eureka Server peers on a single computer, you’ll need to create a virtual host for each Eureka Server peer. Without this, the Eureka cluster won’t function correctly. Now, if each peer is running on a separate computer with its own IP address, you don’t need to define the Eureka Server peers in a hosts file. However, when running all Eureka Server peers on a single computer, it’s necessary to define each peer in a hosts file.
Define Eureka Server Peers on MacOS
If you’re using MacOS, you can define each Eureka Discovery Server peer by editing your hosts file. This file is essentially a map telling your computer where to find the servers identified as ‘peer1’, ‘peer2’, and ‘peer3’. Here’s how you can do it:
First, open a terminal window. You can do this by going to Applications > Utilities > Terminal.
Next, you’ll need to edit the hosts file, which is found at /etc/hosts. We’ll use the built-in text editor “nano” for this purpose. Type the following command into your terminal and press enter:
sudo nano /etc/hosts
You’ll be asked for your password. Go ahead and enter it.
This will open the hosts file in the nano editor. At the end of the file, you’re going to define each Eureka Discovery Server peer with its own line. You’ll be associating each peer name with the local IP address (127.0.0.1), like so:
127.0.0.1 peer1 127.0.0.1 peer2 127.0.0.1 peer3
Each line tells your computer to direct any requests for ‘peer1’, ‘peer2’, or ‘peer3’ to your local machine (127.0.0.1).
Once you’ve entered these lines, save your changes by pressing ‘Control+X’, then ‘Y’ to confirm, and finally ‘Enter’ to write the changes to the file.
And that’s it! You’ve successfully defined each Eureka Discovery Server peer on your MacOS system. Remember, this step is crucial if you’re running all Eureka Server peers on a single computer.”
Define Eureka Server Peers on Windows
To define Eureka Server peers on Windows OS you’ll need to open the Notepad application as an administrator. This is necessary because the hosts file is a system file and needs administrative privileges to edit. To do this, click on the Start button, type ‘Notepad’ in the search box, right-click on the Notepad app in the search results, and select ‘Run as administrator’.
Once the Notepad application is open, you’ll need to open your hosts file. Go to File > Open, and in the File name box, type in C:\Windows\System32\Drivers\etc\hosts
, then click ‘Open’. This will open up your hosts file in Notepad.
At the end of the file, you’ll define each Eureka Discovery Server peer, each on its own line. You’ll associate each peer name with the local IP address (127.0.0.1), like so:
127.0.0.1 peer1 127.0.0.1 peer2 127.0.0.1 peer3
Each line is instructing your computer to direct any requests for ‘peer1’, ‘peer2’, or ‘peer3’ to your local machine (127.0.0.1).
Once you’ve entered these lines, save your changes by clicking on File > Save.
Congratulations! You’ve successfully defined each Eureka Discovery Server peer on your Windows 11 system. As with MacOS, this step is critical if you’re running all Eureka Server peers on a single computer.
Configuring Eureka Server Peer 1
To get my Eureka Server Spring Boot project running as Peer 1, I will create a distinct Spring Boot profile for it, aptly named “peer1”. Create a new file called application-peer1.properties.
Why is the file named application-peer1.properties? In Spring Boot, we can use profiles to segregate parts of our application configuration and make it available only in certain environments. The profile-specific properties in an application are activated via naming convention: application-{profile}.properties
. In our case, “peer1” is the profile we’re setting up, hence the file is named “application-peer1.properties”. This file will contain all the specific configuration for the “peer1” instance of our Eureka Server.
application-peer1.properties
server.port=8761 eureka.instance.hostname=peer1 eureka.client.serviceUrl.defaultZone=http://peer2:8762/eureka,http://peer3:8763/eureka
Let’s break down what each line in this file does:
server.port=8761
: This line specifies the port number on which our “peer1” Eureka Server will run. In this case, it’s 8761. This is like the door number of our server’s house, allowing other servers and services to find and communicate with it.eureka.instance.hostname=peer1
: This line is giving our Eureka Server instance a name, “peer1”. This is how other servers in our cluster will identify this particular server. It’s like naming your pet so you can call it when you need it.eureka.client.serviceUrl.defaultZone=http://peer2:8762/eureka,http://peer3:8763/eureka
: This line is the heart of peer awareness. It’s telling “peer1” where to find its peers. In this case, it’s providing the addresses of “peer2” and “peer3”. The addresses include the hostname (peer2 or peer3), port number (8762 or 8763), and the path to Eureka (/eureka). So, “peer1” knows where to look for its friends in the cluster.
Configuring Eureka Server Peer 2
Now that we have Peer 1 set up, let’s configure Peer 2. Similar to what we did for Peer 1, we’ll create a separate Spring Boot profile named “peer2”. Consequently, we need to create a new file called application-peer2.properties.
Just as we did before, the name of the file – application-peer2.properties – is determined by the Spring Boot profile we’re setting up. This file will contain all the specific configuration for the “peer2” instance of our Eureka Server.
Here’s what the application-peer2.properties file looks like:
server.port=8762 eureka.instance.hostname=peer2 eureka.client.serviceUrl.defaultZone=http://peer1:8761/eureka,http://peer3:8763/eureka
Now, let’s discuss what each line means:
server.port=8762
: This tells our “peer2” Eureka Server to run on port 8762.eureka.instance.hostname=peer2
: This assigns the name “peer2” to our Eureka Server instance, allowing other servers in our cluster to identify it.eureka.client.serviceUrl.defaultZone=http://peer1:8761/eureka,http://peer3:8763/eureka
: This line is crucial for peer awareness. It tells “peer2” where to find its peers – “peer1” and “peer3”. The addresses include the hostname (peer1 or peer3), port number (8761 or 8763), and the path to Eureka (/eureka).
And there we have it! We’ve successfully configured Peer 2. In the next section, we’ll tackle configuring Peer 3!
Configuring Eureka Server Peer 3
Having set up Peer 1 and Peer 2, let’s move on to the configuration of Peer 3. As with the previous peers, we’ll create a dedicated Spring Boot profile named “peer3”. This requires creating a new file named application-peer3.properties.
The name of this file – application-peer3.properties – follows the same pattern as before, determined by the Spring Boot profile we’re creating. This file will contain all the specific configuration for the “peer3” instance of our Eureka Server.
Here’s the content of the application-peer3.properties file:
server.port=8763 eureka.instance.hostname=peer3 eureka.client.serviceUrl.defaultZone=http://peer1:8761/eureka,http://peer2:8762/eureka
Let’s break down what each line of this file means:
server.port=8763
: This line directs our “peer3” Eureka Server to run on port 8763.eureka.instance.hostname=peer3
: This assigns the name “peer3” to our Eureka Server instance, allowing it to be identified by other servers in our cluster.eureka.client.serviceUrl.defaultZone=http://peer1:8761/eureka,http://peer2:8762/eureka
: This line is essential for peer awareness. It informs “peer3” of the locations of its peers – “peer1” and “peer2”. The addresses include the hostname (peer1 or peer2), port number (8761 or 8762), and the path to Eureka (/eureka).
Great job! You’ve now successfully configured Peer 3.
Configure a Shared Eureka Server Application Name
To operate as part of a single cluster, each Eureka Server – or “peer” – needs to share the same application name. This is crucial because each Eureka peer is essentially a replica of the others. Therefore, every replica or peer must have the same application name to effectively operate together as a cohesive unit within the cluster.
In our setup, each peer is configured within its own distinct Spring Boot profile properties file. However, it’s possible for these peers to inherit shared configuration properties. We achieve this by defining these shared properties in a universal application.properties file.
application.properties
spring.application.name=Eureka-Discovery-Server
The line above sets a shared application name “Eureka-Discovery-Server” across all peers. By defining it in the application.properties file, this name is applied to all profiles unless explicitly overridden. This ensures that our Eureka Server peers are identified as part of the same application, promoting efficient and effective communication within our Eureka Server cluster.
Run Eureka Server Cluster with Peer Awareness
We are now ready to run our Eureka Server Cluster. It is a single Spring Boot application with three distinct Spring Boot profiles. Each profile corresponds to a different peer in our cluster – peer1, peer2, and peer3 – each with their own unique configurations.
To run the Eureka Server cluster, we need to start our Spring Boot application three times, once for each profile. Here’s how to do it:
- Open a terminal window. Navigate to the directory where your project is located using the
cd
command. For example, if your project is in a folder named “myproject” on your desktop, you would type:cd Desktop/myproject
. - Run the first instance of the Eureka Server for “peer1” using the following command:
mvn spring-boot:run -Dspring-boot.run.profiles=peer1
- Open a second terminal window. Again, navigate to your project’s directory.
- Run the second instance of the Eureka Server for “peer2” using the command:
mvn spring-boot:run -Dspring-boot.run.profiles=peer2
- Open a third terminal window and navigate to your project’s directory.
- Finally, run the third instance of the Eureka Server for “peer3” using the command:
mvn spring-boot:run -Dspring-boot.run.profiles=peer3
Each command above runs the Eureka Server with a specific profile. The -Dspring-boot.run.profiles
flag is used to specify which Spring Boot profile to use when running the application. By running these commands in separate terminal windows, we’re launching three instances of our Eureka Server, each configured with a different profile, forming our Eureka Server cluster.
That’s it! You’ve now successfully run your Eureka Server Cluster. Each Eureka Server is aware of its peers and can share instances registered with them. This makes your microservices more resilient and fault-tolerant. Well done!
Viewing the Eureka Server Dashboards
After successfully running our Eureka Server cluster, we can now view the Eureka Server dashboard for each peer. The Eureka Server dashboard provides a user-friendly interface that allows us to monitor the registered microservices, their status, and other useful information.
To access the dashboard for each Eureka Server peer, follow the steps below:
- Open a web browser of your choice.
- In the address bar, type in the hostname and port number of the Eureka Server peer you want to view. Each peer runs on a different port, as we configured in the earlier steps. The hostnames “peer1”, “peer2”, and “peer3” correspond to the local address 127.0.0.1 (localhost) that we defined in our hosts file.
- For Peer 1, type
http://peer1:8761
- For Peer 2, type
http://peer2:8762
- For Peer 3, type
http://peer3:8763
- For Peer 1, type
- Press Enter. You should now see the Eureka Server dashboard for the respective peer. It will display a list of registered microservices, their status, and other details.
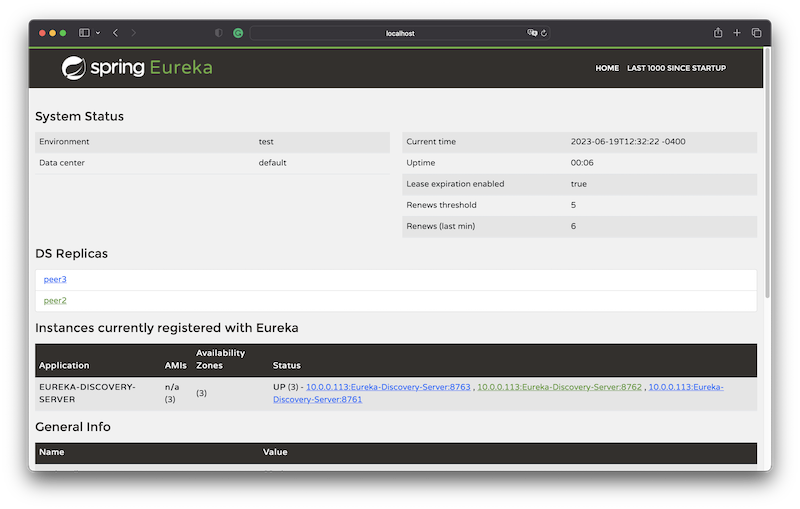
Remember, each Eureka Server peer has its own dashboard, reflecting its view of the registered services. The dashboards might differ slightly between peers due to the nature of distributed systems, where changes (like service registrations and de-registrations) take a short while to propagate through the entire cluster.
By inspecting these dashboards, you can verify that your Eureka Server cluster with Peer Awareness is functioning correctly, with each peer aware of the other peers and the services registered with them. This offers a valuable tool for monitoring the health and status of your microservices.
Register Eureka Client with Eureka Server Cluster
So, how does a Eureka Client – one of your microservices – register with this cluster? It’s pretty straightforward, actually. The client needs to know the addresses of all the Eureka Servers in the cluster. It gets these addresses from a configuration file in your application, typically named application.properties
.
In your application.properties
file, you might have a line that looks something like this:
spring.application.name=discovery-client eureka.client.serviceUrl.defaultZone=http://peer1:8761/eureka,http://peer2:8762/eureka,http://peer1:8763/eureka
This line is telling your Eureka Client where to find the Eureka Servers. The part after the equals sign (=
) lists the addresses of the servers. Each address is separated by a comma (,
).
In this example, we have three Eureka Servers, each running on a different port (8761, 8762, and 8763) on the hosts named peer1
and peer2
. The /eureka
at the end of each URL is the path where the Eureka Server is listening for connections.
When your Eureka Client starts up, it reads this configuration and tries to register itself with the first Eureka Server in the list. If that server is unavailable, it attempts to register with the next one, and so on. Once your client is registered with a server, that Eureka Server shares its registry with the other servers in the cluster. So even though your client registers with one server, its information is available throughout the cluster. This ensures that even if one server goes down, your client continues to be discoverable by other microservices in your application.
And that’s it! You’ve now learned how to make a Eureka Client register with a Eureka Server cluster. It’s a key step to creating robust, resilient microservices architectures. Keep practicing, and you’ll get the hang of it in no time.
Troubleshooting
If your Eureka Server cluster doesn’t seem to be operating as expected, don’t worry. Here are some common issues and suggestions on how to resolve them.
- Check the Main Application File: Ensure that you have annotated your main application file with the @EnableEurekaServer annotation. This annotation is crucial as it enables the Eureka Server capabilities in your Spring Boot application. Without it, your application won’t function as a Eureka Server.
- Verify Hosts File Configuration: If you are running the Eureka Server cluster on your local machine, make sure that you have assigned a unique hostname to each Eureka peer in your “hosts” file. Remember, the hostnames help the peers to locate each other.
- Consistent Application Name: It’s important that all your Eureka peers use the same ‘spring.application.name’. This allows each Eureka peer to recognize others as part of the same cluster, ensuring that they can replicate their registries between each other correctly.
- Unique and Available Port Number: Check that each Eureka Server peer is running on a unique and available port number. Overlapping port numbers or trying to use a port that is already in use by another process can prevent a peer from starting up properly.
- Correct Service URLs: Confirm that the ‘eureka.client.serviceUrl.defaultZone’ property points to the correct hostnames and port numbers of the other Eureka Server peers. This property tells each peer where to locate its companions in the cluster.
- Accessing the Eureka Dashboards: When trying to access the Eureka Server dashboard in your browser, ensure you’re using the correct port number for the peer you want to view. Remember, the URL is the hostname followed by the port number, like so: ‘http://peer1:8761‘. There’s no need to add ‘/eureka’ at the end when accessing the dashboard.
Remember, troubleshooting is a normal part of any development process. Take one step at a time, and you’ll have your Eureka Server cluster up and running in no time!
Final Words
Congratulations! You’ve successfully set up and run your Eureka Server Cluster with peer awareness. This tutorial provided detailed steps to configure your Eureka servers, run the cluster with different profiles, and view the Eureka Server dashboard for each peer. Remember, the key to a robust Eureka cluster lies in the proper configuration and understanding of each peer’s role.
If you face any issues, don’t forget to refer to the “Troubleshooting” section. It covers common issues that you might encounter and their solutions. Keep in mind that community and online resources are your friends when dealing with complex software setups.
Lastly, always remember that learning is a continuous journey. If you’re interested in delving deeper into the world of Spring Cloud and microservices, I highly recommend visiting my Spring Cloud tutorials on the Apps Developer Blog. There, you will find a wealth of resources dedicated to Spring Cloud and a wide range of tutorials for beginners and advanced learners alike.
Thank you for following along with this tutorial. Happy coding, and I look forward to seeing you in future tutorials!